Read Current and Next Line in Python From Text
To read a text file in Python:
- Open the file.
- Read the lines from the file.
- Shut the file.
For example, allow's read a text file chosen example.txt from the same binder of the code file:
with open('example.txt') as file: lines = file.readlines()
At present the lines variable stores all the lines from example.txt as a list of strings.
This is the quick answer. To learn more details about reading files in Python, please read along.
Reading Text Files in Python
Reading a text file into your Python programme follows this procedure:
- Open the file with the built-in open() function past specifying the path of the file into the call.
- Read the text from the file using i of these methods: read(), readline(), readlines().
- Close the file using the shut() method. You can permit Python handle closing the file automatically by opening the file using the with argument.
one. How to Use the open up() Function in Python
The basic syntax for calling the open up() office is:
open(path_to_a_file, mode)
Where:
- path_to_a_file is the path to the file you want to open. For example Desktop/Python/example.txt. If your python program file is in the same folder as the text file, the path is just the name of the file.
- mode specifies in which state yous want to open the file. There are multiple options. But as you're interested in reading a file, yous but need the way 'r'.
The open up() function returns an iterable file object with which you can hands read the contents of the file.
For instance, if you have a file called case.txt in the same folder equally your code file, y'all can open it by:
file = open up("example.txt", "r")
Now the file is opened, but not used in whatever useful way even so.
Next, let's accept a look at how to actually read the opened file in Python.
two. File Reading Methods in Python
To read an opened file, let'due south focus on the iii different text reading methods: read(), readline(), and readlines():
- read() reads all the text from a file into a single string and returns the string.
- readline() reads the file line by line and returns each line as a separate string.
- readlines() reads the file line by line and returns the whole file as a list of strings, where each string is a line from the file.
Later you are going to see examples of each of these methods.
For instance, let's read the contents of a file called "example.txt" to a variable as a string:
file = open("example.txt") contents = file.read()
Now the contents variable has whatever is within the file as a single long cord.
3. Ever Close the File in Python
In Python, an opened file remains open every bit long as you don't close information technology. Then make sure to close the file after using it. This can be done with the close() method. This is important because the program tin can crash or the file tin corrupt if left hanging open up.
file.close()
You tin also let Python take care of closing the file by using the with argument when dealing with files. In this example, you don't need the close() method at all.
The with statement structure looks like this:
with open(path_to_file) every bit file: #read the file here
Using the with statement is and then convenient and conventional, that we are going to stick with it for the rest of the guide.
Now yous understand the basics of reading files in Python. Next, allow's have a wait at reading files in action using the different reading functions.
Using the File Reading Methods in Python
To echo the following examples, create a folder that has the post-obit two files:
- A reader.py file for reading text files.
- An example.txt file from where your program reads the text.
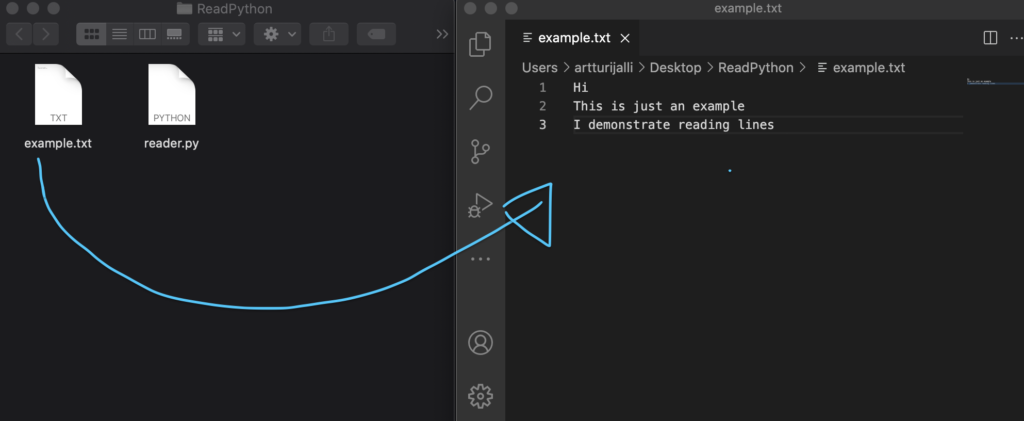
Also, write some text on multiple lines into the case.txt file.
The read() method in Python
To read a file to a single string, apply the read() method. Every bit discussed above, this reads whatever is in the file as a single long cord into your plan.
For example, let's read and print out the contents of the instance.txt file:
with open("example.txt") as file: contents = file.read() print(contents)
Running this piece of code displays the contents of instance.txt in the console:
Hi This is just an instance I demonstrate reading lines
The readline() Method in Python
To read a file i line at a time, use the readline() method. This reads the current line in the opened file and moves the line pointer to the next line. To read the whole file, use a while loop to read each line and motion the file pointer until the end of the file is reached.
For example:
with open('example.txt') as file: next_line = file.readline() while next_line: print(next_line) next_line = file.readline()
As a consequence, this program prints out the lines one by one as the while loop proceeds:
Hullo This is but an example I'm demonstrate reading lines
The readlines() Method in Python
To read all the lines of a file into a list of strings, use the readlines() method.
When you have read the lines, yous can loop through the listing of lines and print them out for example:
with open('example.txt') equally file: lines = file.readlines() line_num = 0 for line in lines: line_num += 1 print(f"line {line_num}: {line}")
This displays each line in the console:
line 1: Hi line 2: This is but an case line 3: I'm demonstrate reading lines
Use a For Loop to Read an Opened File
You just learned almost three unlike methods you can use to read a file into your Python plan.
It is skillful to realize that the open() function returns an iterable object. This means that you tin can loop through an opened file just similar y'all would loop through a list in Python.
For example:
with open up('instance.txt') equally file: for line in file: impress(line)
Output:
Hi This is simply an case I'm demonstrate reading lines
As you lot can meet, you did not demand to use whatsoever of the congenital-in file reading methods. Instead, you used a for loop to run through the file line by line.
Conclusion
Today you learned how to read a text file into your Python plan.
To recap, reading files follows these three steps:
- Use theopen() function with the'r' mode to open a text file.
- Use one of these iii methods:read(),readline(), orreadlines() to read the file.
- Close the file later on reading it using theshut() method or let Python do information technology automatically past using thewith statement.
Conventionally, yous can also use the with argument to reading a file. This automatically closes the file for you and so you do not need to worry about the third step.
Cheers for reading. Happy coding!
Farther Reading
Python—How to Write to a File
10 Useful Python Snippets to Code Like a Pro
Source: https://www.codingem.com/read-textfile-into-python-program/
0 Response to "Read Current and Next Line in Python From Text"
Postar um comentário